This one is broken because I am not looping through the fields to get the correct object set up and I had hard coded the Dictionary entry with the index “TestText”
Me – yesterday
I am also seeing that my test isn’t actually doing what I want.
The test is using the functions to change the fields, but the functions each save the form by setting Form.Dirty = False after each change. Access is going to run multiple BeforeUpdate Events for the form, one for each Form.Dirty = False. So I will need to change my function. Rather than refactor anything outside my test, I’ll just update the test code directly to make sure I’m doing this and I can refactor later.
Here’s my full test function before the change:
I am going to change these lines in the middle:
And now the full test function looks like this:
I updated the code within the added With block. This ensures the starting values, sets up the auditor to start listening to changes, then changes and saves the fields in one save. This will still fail because there won’t be a TestCombo entry yet due to my FormAuditor object not checking multiple fields. So now I’ll take a look at that function.
Oops, forgot to update the field lines above with an = operator, which I have now done, but the compiler caught me on it.
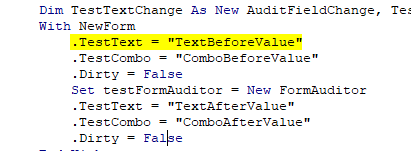
Now, my code inside the BeforeUpdate event is only checking for one field and adding one item to the collection:
But I need to loop through the controls and check multiple controls to see if they’ve changed so let’s add a loop to loop through all the controls:
Now I have a loop and I updated the Field Changed to use an Access Control type object and am building the dictionary of changes through the loop. I had to modify things a bit because I was calling the CreateAuditEventDetails multiple times in the loop and I only want to call that once per BeforeUpdate Event. Next time I should be able to finish this off by updating the CreateAuditEventDetails to accept a FieldChanges dictionary and return the object.