Starting with this non-passing test:
'@TestMethod("Verify Changes")
Private Sub WhenTextFieldChangesBeforeAndAfterValuesAreReturned()
Dim testFormAuditor As FormAuditor
Dim testCollection As New Collection
ChangeTestText "BeforeValue"
Set testFormAuditor = New FormAuditor
ChangeTestText "AfterValue"
Set testCollection = testFormAuditor.ListOfChanges
With testCollection.Item(1).FieldChanges("TestText")
Assert.IsTrue .OldValue = "BeforeValue" And .NewValue = "AfterValue"
End With
End Sub
Currently the Collection returned by ListOfChanges is only a text string of what TestText changed to. But after yesterday, I have 2 new objects to use in my dictionary and collection to store the changes to the field and refer to it by name. So let’s take a look at the code in the BeforeUpdate method hook in the FormAuditor class that needs to build this item to add to the collection:
Private Sub FormToAudit_BeforeUpdate(Cancel As Integer)
If FieldChanged(FormToAudit.TestText) Then pListOfChanges.Add FormToAudit.TestText.Value
End Sub
All right, that looks easy enough. I just need to change the FormToAudit.TestText.Value into the object I want to return. I think I’ll throw in a function here to return a AuditEventDetails object:
Private Sub FormToAudit_BeforeUpdate(Cancel As Integer)
If FieldChanged(FormToAudit.TestText) Then pListOfChanges.Add CreateAuditEventDetails
End Sub
Private Function CreateAuditEventDetails() As AuditEventDetails
Dim retVal As AuditEventDetails
Set retVal = New AuditEventDetails
retVal.FieldChanges.Add "TestText", CreateAuditFieldChange("TestText")
Set CreateAuditEventDetails = retVal
End Function
Private Function CreateAuditFieldChange(FieldName As String) As AuditFieldChange
Dim retVal As AuditFieldChange
Set retVal = New AuditFieldChange
retVal.OldValue = FormToAudit(FieldName).OldValue
retVal.NewValue = FormToAudit(FieldName).Value
Set CreateAuditFieldChange = retVal
End Function
And of course once I started creating the AuditEventDetails object, I needed an AuditFieldChange object function to create an instance of that. Ok, that might work. I’ll run the test.
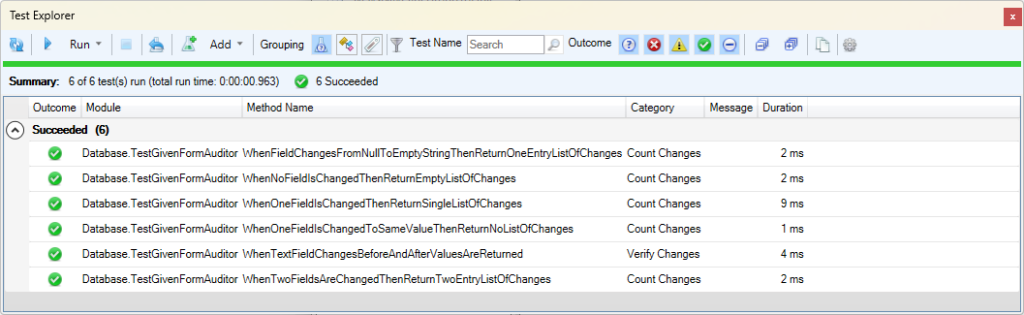
Sweet! That worked. Ok, so next we have to get this working in cases of multiple fields and multiple changes. We’re going to need loops over controls and all sorts of fun things. This is where it’s finally going to start getting exciting, which is very apropos since this is article 42! You do know that 42 is the answer to the question of life, the universe, and everything, right?