Now our auditor will need to be a little smarter. We need to check the beginning and ending value of the test text field during the test in the form Before Update event. We won’t yet consider canceled BeforeUpdate events, I’ll just keep that in the back of my mind for later. Right now we have a failing test that needs to pass.
Here is the Test code again:
The test is just setting the TextBox string back to itself.
Here is our FormAuditor class so far:
So it looks like I could do the check in the BeforeUpdate event at the bottom like this:
I think in this case this will pass the test. Let’s see!
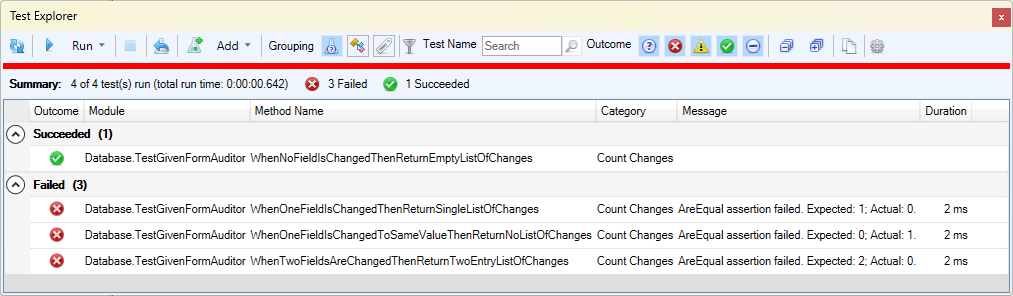
Uh oh. I now have 3 broken tests. Looking at the expected results vs the actual, they look backwards. Looks like I reversed the condition I wanted and only am adding to the list of changes when the old value and new value are the SAME! Well that’s easy to fix:
That’s better, I bet it will work now:
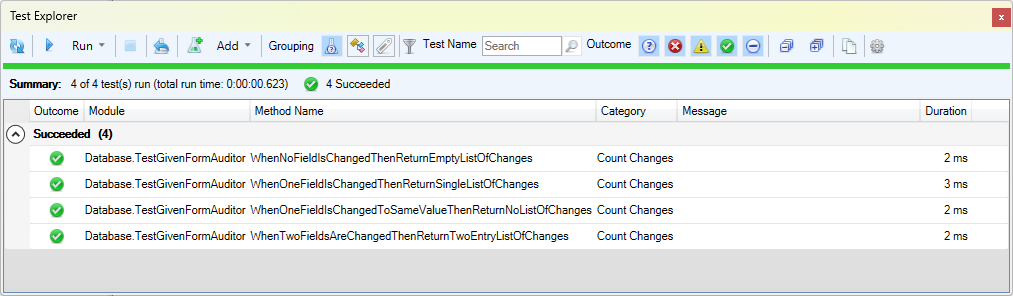
There we go. Now I’ll forgo refactoring for now and see if I can think up another requirement I want to prove is currently failing. I think my comparison is somewhat weak. Let’s test the null values cases next.
I just played around a little with the Form and null using the Intermediate window.
It seems doing a If Null = “” condition does not fail but returns false.
My little function ChangeTestText though will not accept a Null, so the test itself would fail if I tried to set the control value to Null using the function. Do I want the Auditor to make sure that if the control is empty with Null or empty with a 0 length string it treats them the same? Or do I want the Auditor to do strict typing? Hmmm… sounds like a question for another day.