It is time to think about the next test. What I’d like to do is start capturing changes to the form fields. Probably the simplest and easiest way to do that is to create a collection of form field information inside the FormListener object to start storing changes made to that field.
The easiest thing I can think of is to have a test box on the test form which we already do, change the field again as we did in a previous test, and then return a count of the times that field changed.
I think I will want either a method or a property on the listener to return the number of times the field was changed. Our first test will simply be to test the field changing from nothing to something.
I will use a method because I want to pass the field name. I think this will all probably change in the future, but that’s ok, I’m just going for as simple as possible.
So my test will be something like this:
So now I’ve added that test and it’s time to watch it fail miserably. So I run the tests and get this error:
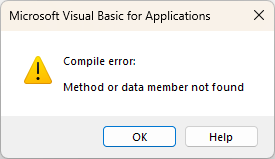
Which makes sense because I have not yet added the “TimesFieldChanged” method to the FormListener object.
So let’s add that method and just make it return 1. That will allow the test to pass.
I’ve used a descriptive name for the method (function) and the variable we must pass in. I’ve set the return value to Long so we can return super huge counts if needed. Billions and more. I don’t know how many times something will change.
When I write functions in Visual Basic for Applications, I usually use a stand-in variable for portability of the code functionality. In other words, let’s say I change the name of the function, I don’t want to have to find more than one spot to change the return statement. VBA requires the use of the name of the function itself to set the return value. I’d prefer if it just allowed us to use a Return statement like other languages I’ve used, but it doesn’t, so I do this to make it easier on myself when I change function names or copy code to another function.
Unsurprisingly, the test now passes. Uh, never mind:
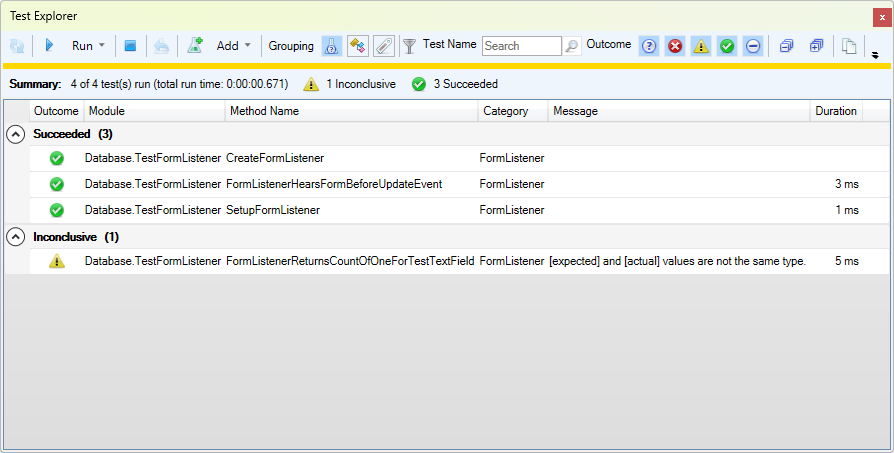
So, in order for the test to see “1” as a Long, I will need to change my test code. I’ll do that next time.